This is a web site i created at my lab in order to automate Cisco ACI configuration via REST API
For example: Configuring server port:
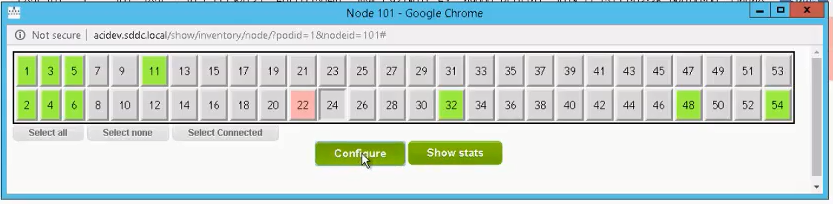
The web site is running in Ubuntu server with Apache2 and was developed in PHP using NetBeans IDE
_
–
A demo:
–
The source code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 |
<?php $global_apic_url="https://apic1.sddc.local:443/api/"; $global_apic_user="admin"; $global_apic_pass="XXXXX"; $token_file_path="/var/www/html/include/token.txt";// Apic Token function read_token(){ global $token_file_path; $token_file = fopen($token_file_path, "r"); $token= fread($token_file,filesize($token_file_path)); fclose($token_file); return $token; } function save_token($token){ global $token_file_path; $token_file = fopen($token_file_path, "w"); fwrite($token_file, $token); fclose($token_file); } function aci_connect(){ global $global_apic_url, $token_file_path, $global_apic_user, $global_apic_pass; $url=$global_apic_url."aaaLogin.json"; $data = '{ "aaaUser":{ "attributes":{ "name":"'.$global_apic_user.'", "pwd":"'.$global_apic_pass.'" } } }'; $ch = curl_init($url); curl_setopt($ch, CURLOPT_POST, 1); curl_setopt($ch, CURLOPT_POSTFIELDS, $data); // Disable SSL certificate check curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, 0); // json curl_setopt($ch, CURLOPT_HTTPHEADER, array('Content-Type: application/json')); curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1); $result_json = curl_exec($ch); if(curl_errno($ch)){ die( 'Curl error: '.curl_error($ch));} curl_close($ch); $result= json_decode($result_json,true); $token= $result["imdata"][0]["aaaLogin"]["attributes"]["token"]; save_token($token); } function aci_get($uri){ global $global_apic_url; $token=read_token(); //print $token; $ch = curl_init($global_apic_url.$uri); curl_setopt($ch, CURLOPT_CUSTOMREQUEST, "GET"); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, 0); curl_setopt($ch, CURLOPT_COOKIE, "APIC-cookie=$token"); $result_json = curl_exec($ch); $result=json_decode($result_json,true); if(curl_errno($ch)){ print 'Curl error: ' . curl_error($ch);} curl_close($ch); // check if need to re-login if (need_to_relogin($result)){ print "## Re-Login ##"; aci_connect(); $result=aci_get($uri); } return $result; } ?> |
–
First we need to find the URI of the REST API call we wants
We can do it via the API inspector
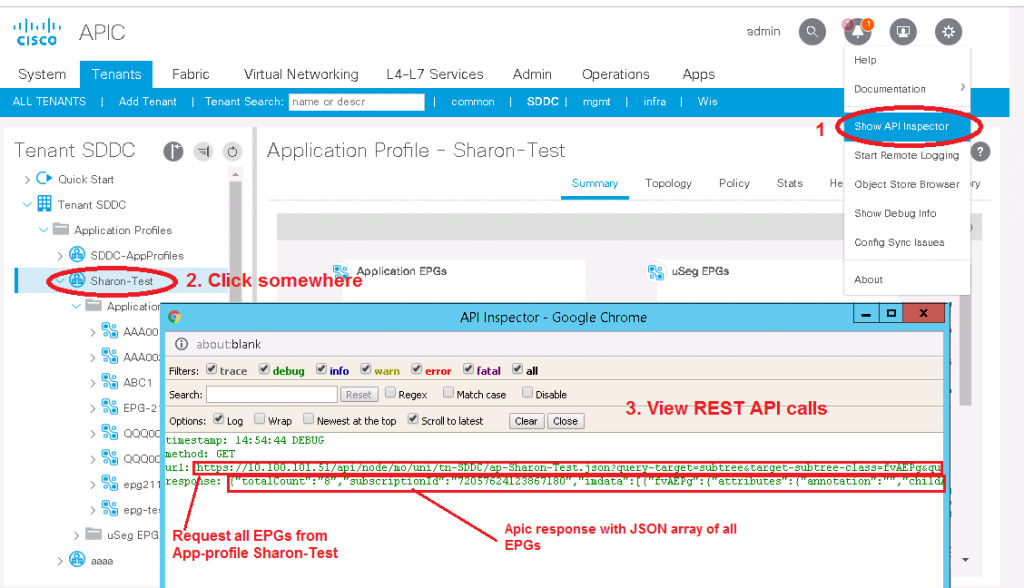
–
Then we can test the code
(for example Get all the VRFs from tanent SDDC) :
1 2 3 4 |
<?php $result= aci_get('node/mo/uni/tn-SDDC.json?query-target=children&target-subtree-class=fvCtx&query-target-filter=not(wcard(polUni.dn, "__ui_"))&order-by=fvCtx.name|asc'); print_r($result); ?> |
Will return an array of VRFs: (The Apic returns JESON, array I convert it to PHP array)
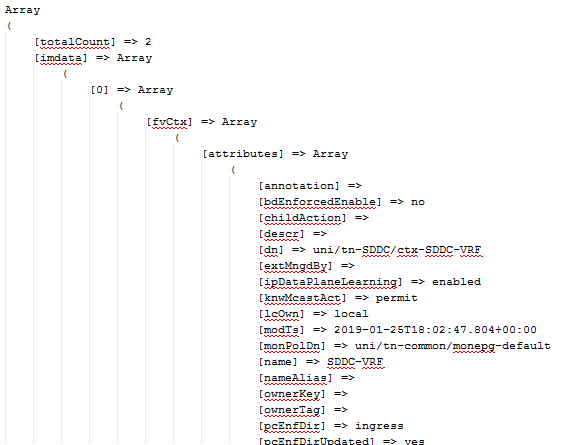
And then i parsed the returned array and showed it at the browser inside a table using HTML
–
–
Configuring the Apic
I prefer to send to the Apic XML data with all the parameters i wants to configure,
the easiest way to find the current format is to save an object from the Apic GUI and open it with Internet explorer (Yes, this is not a mistake :), because IE by default interpreted XML data)
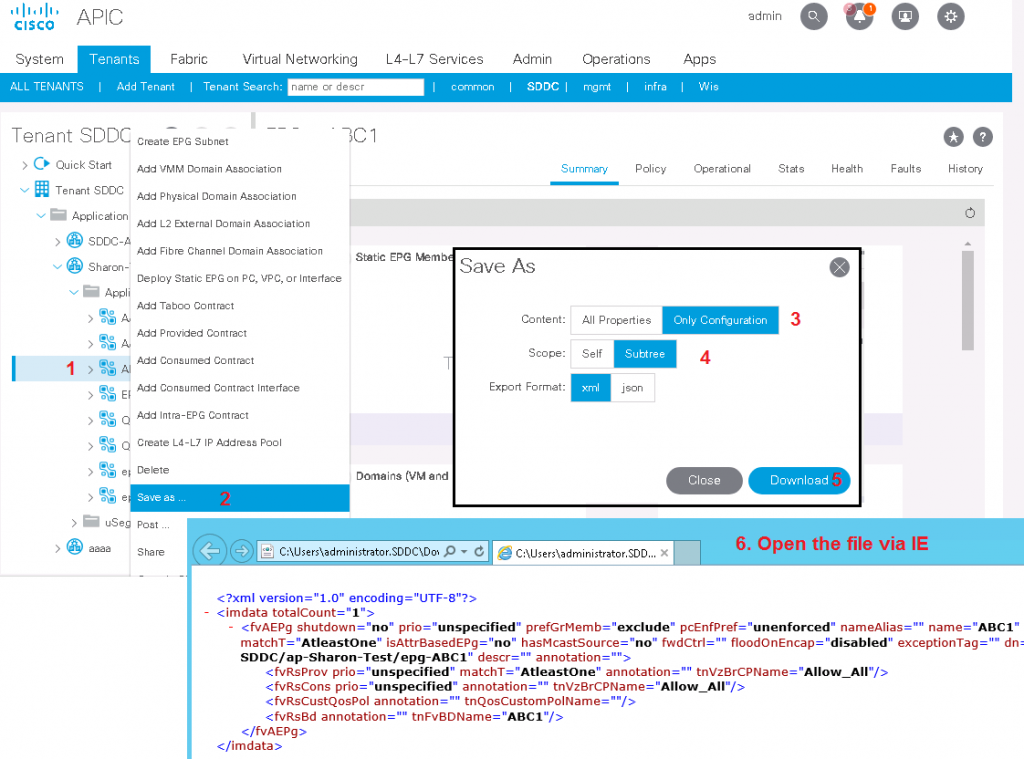
_
Summery
Automation with Cisco ACI is Easy and works good
_
Hi Sharon,
Very nice work, this would make ACI configuration a lot easier for simple tasks.
Would you be willing to share the rest of the code 🙂 or are you planning to make it commercial software and sell it 🙁
Thanks