Few days ago i bought an “old games” emulator, the problem was that all games names was in Chinese although all the menus was in English
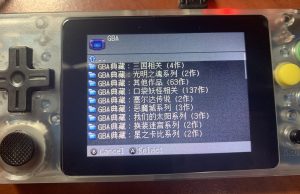
I removed the memory card from the emulator and connected it to my PC, then i saw all games files (.zip) names was in Chinese, so i decided to translate file names to English and hope that the games list will also be in English
I looked for a program to do that in the internet with no luck, so i wrote a small python script to translate using google translate API, Here is how it looked like while the translate was running:
The script:
https://github.com/sharonsa/translate
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 |
from googletrans import Translator import os import sys import glob import shutil import ntpath import time def isEnglish(s): try: s.encode(encoding='utf-8').decode('ascii') except UnicodeDecodeError: return False else: return True translator = Translator() path=u'J:\\roms\\' def walkdirs(path): rename(path) for root, dirs, files in os.walk(path, topdown=True): for dir in dirs: dir_path=path+dir+"\\" print("Moving to dir " +dir_path) sys.stdout.flush() walkdirs(dir_path) def rename(path): files=glob.glob(path + "*") i=0 for file in files: if isEnglish(file): print (file) else: i=i+1 old_name=file try: file_base=ntpath.basename(file) file_arr=os.path.splitext(file_base) file_name=file_arr[0] file_ext=file_arr[1] new_name=ntpath.dirname(file)+ "\\"+translator.translate(file_name).text.replace(":","-") new_name=new_name+"."+file_ext except: print ("Translating error") sys.stdout.flush() i=i-1 pass continue try: print ("renaming "+ str(old_name) +" to "+ str(new_name)) sys.stdout.flush() except: try: print ("renaming XXX to "+ str(new_name)) sys.stdout.flush() except: print ("renaming XXX to YYY") sys.stdout.flush() pass shutil.move(old_name, new_name) time.sleep(.300) print (str(i) +" files translated") walkdirs(path) |
I had few problems:
- Google limits the number of translates per day – you need to change your IP address after something like 500 translates (By restarting your internet router or by using a VPN (the error was – “JSONDecodeError: Expecting value: line 1 column 1 (char 0)“
- Some of the names python even can’t show with print, so i added try to the ‘print’ command
Here is how it looks after:
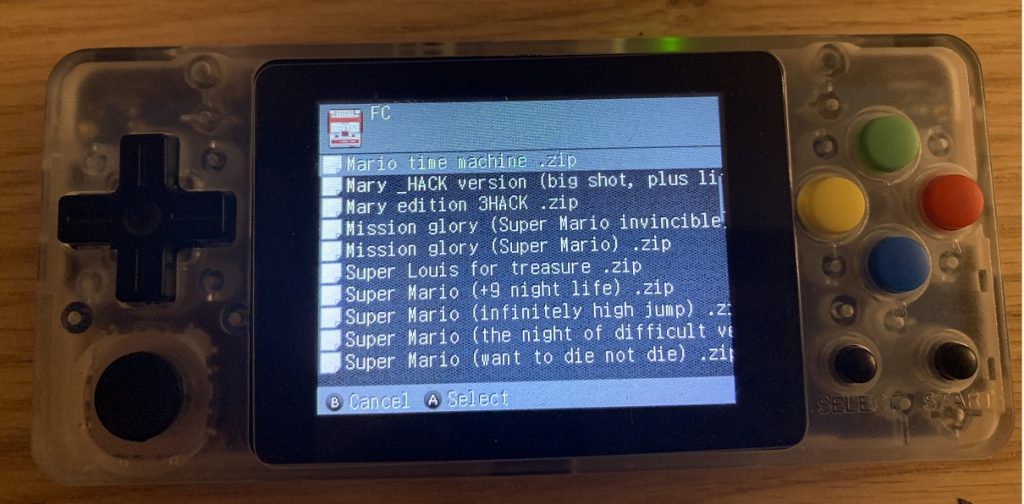
sir how to use this script to translate chinese folders to english
Hi, Its a Python script
You know, a program that can translate file names would be very useful. You should consider it. If I knew Python at all, I’d be making that program to sell.
Muy interesante y muy útil sería tener un programa que pudiera traducir nombres de carpetas y archivos de forma masiva.
Puede explicar o hacer un tutorial de como lograrlo con su script?
Tengo montones de carpetas y archivos que quisiera traducir
Muchas gracias
Thanks for posting this script – very useful.
Couple of comments in-case anyone is having issues:
– if you copy and paste into an IDE be careful as the code contains tabs and space indentation, better to open in a text editor and replace tabs with spaces
– translation may fail due to googletrans library, in which case you can try “pip install googletrans==4.0.0-rc1”
-Further details here:
https://stackoverflow.com/questions/52455774/googletrans-stopped-working-with-error-nonetype-object-has-no-attribute-group
Thanks again.
Can you please post your revised code? I can not correct the issue with the indention.
I just get a lot of translating errors instead of actual translations.
I must be doing something wrong with my code, but I don’t know what. I can hopefully send it to you if you respond.
I am trying to translate Japanese to English. The script run on my folder and says 0 files translated. Any ideas?
I’ve tried to use this script, and it doesn’t work.
I point it to a folder with a lot of Japanese name image files, and it doesn’t translate at all.
It’s not an issue with the indenting, so I imply don’t know.
I would really appreciate help.